· Heybounce · Guides · 6 min read
How to Use Regex for Email Validation (and Why It’s Not Enough)
Regex helps validate email syntax, but it's only one piece of the puzzle for reliable email validation.
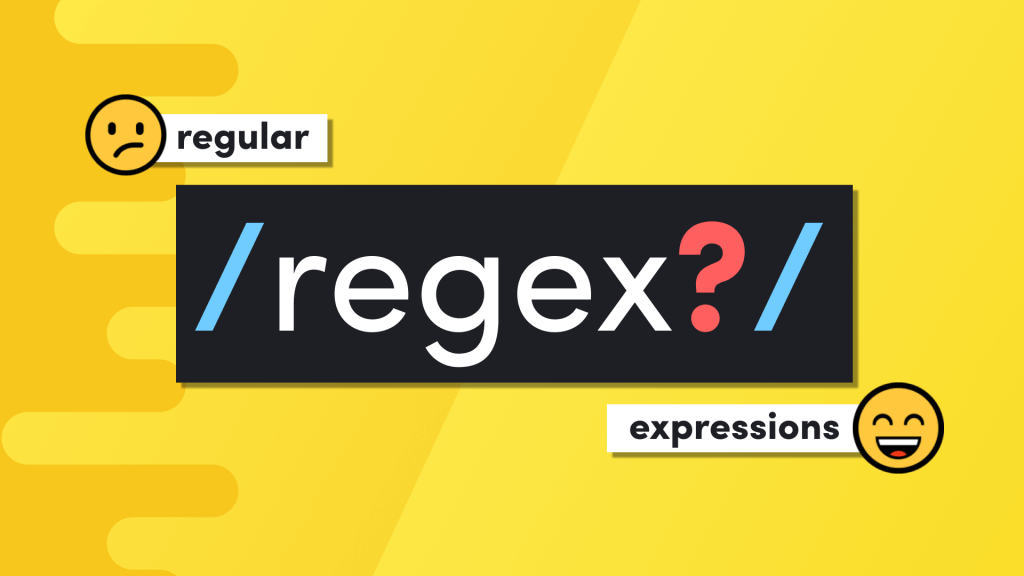
If you’re a developer, you’ve probably used Regular Expressions (Regex) at least once for email validation. Regex can feel like an elegant hammer for a nail that needs validating. After all, email validation is just about checking the structure, right? Well, not quite. While Regex is a powerful tool, there’s a lot more to proper email validation than a neatly crafted pattern.
This post will take you through how to use Regex for email validation, what it can (and can’t) do, and why a comprehensive approach is necessary for a real-world scenario.
What Is Regex and How Does It Work for Email Validation?
Regular Expressions, or Regex, are sequences of characters that form a search pattern. Think of it as a way to specify what kind of text you want to match or find within a given input. When it comes to email validation, Regex can help determine if an email address is formatted correctly based on certain rules.
For example, a simple Regex for email validation might look like this:
const emailRegex = /^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/;
This pattern checks for the following:
- Local Part: A combination of letters, digits, dots, underscores, or other common symbols.
- @ Symbol: The obligatory delimiter between the local and domain parts.
- Domain: A series of letters, numbers, dots, and a valid top-level domain (TLD).
Why Regex Falls Short for Email Validation
On the surface, Regex might seem like a comprehensive way to validate emails, but it’s not without limitations. Here are some reasons why using Regex alone is not enough for real-world email validation:
1. Complex Email Standards
The actual specification for valid email addresses, known as RFC 5321 and RFC 5322, is far more complex than most people realize. Emails like very."odd\@example.com
or user@[192.168.1.1]
are technically valid according to the standard, but they might not match many Regex patterns.
Building a Regex pattern that matches every single possible valid email format is almost impossible. Even if you create an extensive Regex, it tends to get overly complex, hard to maintain, and still can miss the mark.
2. Domain and MX Record Issues
An email address might look valid in structure but still not be usable in practice. Regex doesn’t check whether the domain actually exists or if the domain has MX (Mail Exchange) records configured to receive emails. In other words, [email protected]
could pass a Regex check, but sending an email there would fail because the domain doesn’t exist.
3. User Typos and Common Mistakes
Even if an email matches the Regex pattern, it doesn’t guarantee that it’s the correct address. For example, typos like [email protected]
instead of [email protected]
will still pass a Regex check. These small errors can lead to serious delivery issues down the road if undetected.
Common Regex Patterns for Email Validation
Let’s take a look at some popular Regex patterns used for email validation and their pros and cons.
1. Simple Regex
const simpleRegex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
- Pros: Simple, easy to understand, and effective for the most common email formats.
- Cons: It allows many invalid email formats and does not conform to the complete RFC specification.
2. More Complex Regex
const complexRegex = /^[a-zA-Z0-9.!#$%&'*+/=?^_`{|}~-]+@[a-zA-Z0-9-]+(?:\.[a-zA-Z0-9-]+)*$/;
- Pros: It does a better job covering a wider variety of valid formats.
- Cons: Even a complex Regex like this can still miss edge cases, and the code can be hard to maintain.
The Limits of Regex – Where It Fails
1. Validating Domains
Regex cannot tell if a domain exists or if it’s configured to receive mail. For that, you need to perform a DNS Lookup to see if the domain has valid MX records.
2. Syntax vs. Deliverability
Regex is only good at syntax validation, not deliverability. An email may look correct but may still be undeliverable due to issues like temporary server problems or spam filters.
3. Performance Issues
Complex Regex patterns can be resource-heavy. If you’re processing thousands or millions of emails, Regex validation can become a bottleneck, affecting overall performance.
What Should You Use Alongside Regex for Better Email Validation?
So if Regex alone isn’t enough, what should you do instead? A multi-step validation approach is the answer to reliable email validation.
Here’s a better strategy to validate email addresses:
1. Syntax Validation (Regex)
Start with a simple Regex to quickly filter out clearly malformed email addresses.
2. Domain Verification
Check if the domain of the email address actually exists by performing a DNS lookup. If the domain has MX records, you know there’s at least a server that can theoretically receive emails.
3. Mailbox Verification
Perform an SMTP check to verify if the mailbox really exists. This involves pinging the mail server to confirm that the specific email address is active and capable of receiving messages.
4. Temporary and Disposable Email Checks
There are services that help identify disposable email addresses (DEA) like those provided by 10minutemail.com
. These addresses often lead to poor data quality, so filtering them out can be crucial.
5. Typo Detection
Use services or algorithms that can detect common typos (e.g., gamil.com
instead of gmail.com
). This is a great way to improve data quality and avoid undeliverable emails.
Practical Regex Examples: Email Validation in Different Languages
To make it easier for developers, here are some practical examples of using Regex for email validation in popular programming languages:
1. JavaScript
function validateEmail(email) {
const regex = /^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/;
return regex.test(email);
}
2. Python
import re
def validate_email(email):
regex = r'^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$'
return re.match(regex, email)
3. PHP
function validateEmail($email) {
return filter_var($email, FILTER_VALIDATE_EMAIL) !== false;
}
While these examples use Regex, remember that they only check if the email is formatted correctly and do not guarantee deliverability.
Regex: Friend, But Not a Standalone Solution
So where does that leave Regex? It’s a useful tool in your email validation toolkit, but it’s far from a complete solution. The best approach combines multiple strategies, including:
- Syntax checks with Regex.
- Domain and DNS verification.
- Mailbox-level verification.
Combining these methods ensures that emails are both valid in format and deliverable, which is critical for high-quality email data.
Key Takeaways
- Regex is great for initial syntax checks, but it’s not perfect and can miss valid addresses.
- Domains must be verified through DNS to check if they actually exist.
- SMTP validation is essential to verify that the mailbox is live and capable of receiving messages.
- Typo detection and disposable email detection add an extra layer of accuracy.
Ready to Improve Your Email Validation?
If you’re tired of Regex-only solutions and need something that covers all the bases, give Heybounce a shot. Clean email lists mean better deliverability, improved sender reputation, and ultimately, more effective communication. Start using Heybounce now and see the difference it makes!