· Heybounce · Guides · 5 min read
Ultimate Guide To Validating Emails With Regex
Learn how to use regex for email validation across different programming languages and ensure your email campaigns are effective by eliminating invalid addresses.
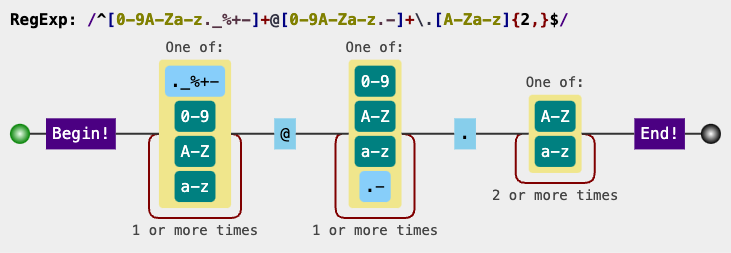
In the world of email marketing, keeping your contact lists clean is vital to ensuring successful campaigns. Invalid email addresses can lead to increased bounce rates, harming your sender reputation and potentially leading to blacklisting. If you’re a developer or a business professional managing an email verification process, you’ve likely heard of using Regular Expressions (regex) for email validation. In this blog, we’ll break down how regex works and why it’s useful for email validation.
What is Regex?
Regex, short for Regular Expressions, is a sequence of characters that forms a search pattern. It’s a powerful tool used for text matching and is highly valuable for developers when handling form submissions. In the context of email validation, regex helps identify whether a given string follows the standard email address format. This can help prevent invalid addresses from entering your database, saving you from future headaches.
Using regex to validate emails allows for the elimination of common typos and formatting issues like missing “@” signs, incorrect domain names, or spaces. This simple layer of validation ensures that only properly formatted email addresses get through, which helps maintain a healthier list for your B2B outreach.
The Anatomy of an Email Regex Pattern
Email addresses have specific rules they must follow. They typically consist of three main components:
- The local part: This is the part before the “@” symbol, which often contains letters, numbers, periods, and underscores.
- The @ symbol: This is a constant that separates the local part from the domain.
- The domain: The part after the “@”, which must include at least one period, representing the top-level domain (TLD), such as “.com”, “.net”, or “.io”.
A typical regex pattern for validating an email address might look like this:
^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$
Let’s break down what each part of this pattern does:
^[a-zA-Z0-9._%+-]+
– This part ensures that the local part of the email contains acceptable characters, including letters, numbers, and a few special characters.@
– The “@” symbol is required and acts as a separator.[a-zA-Z0-9.-]+
– This part allows letters, numbers, periods, and hyphens in the domain name.\.[a-zA-Z]{2,}$
– Finally, the domain must end with a valid top-level domain (TLD), which is typically between 2 and 6 characters long (like “.com” or “.io”).
Limitations of Regex for Email Validation
While regex is great for a preliminary check, it does have its limitations. Regex can only validate the format of an email address, not whether it’s deliverable. For instance, an email like [email protected]
could pass a regex check but still not be a valid address in reality.
Code Examples of Using Regex for Email Validation in Code
To give you a sense of how email regex might be implemented in a real application, here are some code examples in different programming languages:
JavaScript
function validateEmail(email) {
const regex = /^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/;
return regex.test(email);
}
const email = '[email protected]';
console.log(validateEmail(email)); // Returns true if the email is valid
Python
import re
def validate_email(email):
regex = r'^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$'
return re.match(regex, email) is not None
email = "[email protected]"
print(validate_email(email)) # Returns True if the email is valid
PHP
function validateEmail($email) {
$regex = '/^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/';
return preg_match($regex, $email) === 1;
}
$email = "[email protected]";
echo validateEmail($email) ? 'Valid' : 'Invalid'; // Prints 'Valid' if the email is valid
Java
import java.util.regex.*;
public class EmailValidator {
public static boolean validateEmail(String email) {
String regex = "^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\\.[a-zA-Z]{2,}$";
Pattern pattern = Pattern.compile(regex);
Matcher matcher = pattern.matcher(email);
return matcher.matches();
}
public static void main(String[] args) {
String email = "[email protected]";
System.out.println(validateEmail(email)); // Returns true if the email is valid
}
}
Go
package main
import (
"fmt"
"regexp"
)
func validateEmail(email string) bool {
regex := `^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$`
re := regexp.MustCompile(regex)
return re.MatchString(email)
}
func main() {
email := "[email protected]"
fmt.Println(validateEmail(email)) // Returns true if the email is valid
}
Rust
use regex::Regex;
fn validate_email(email: &str) -> bool {
let regex = Regex::new(r"^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$").unwrap();
regex.is_match(email)
}
fn main() {
let email = "[email protected]";
println!("{}", validate_email(email)); // Returns true if the email is valid
}
Conclusion: Regex and Beyond for Effective Email Verification
Regex is a powerful, fundamental tool for email validation, helping catch basic formatting errors and keeping invalid addresses out of your system. However, in a B2B environment, simply checking the format is not enough. Comprehensive email verification takes email validation further, combining syntax validation with deliverability checks to give you peace of mind and a higher ROI on your email campaigns.
If you’re ready to supercharge your email marketing efforts and reduce bounce rates, Heybounce can help. Heybounce not only checks the syntax but also validates whether the domain exists, whether the mailbox is active, and if the email can successfully receive messages. This comprehensive verification ensures that your email campaigns reach real, active inboxes.
The Advantages of Using Heybounce Over Manual Regex Validation
- Syntax Verification: Heybounce ensures that all addresses conform to a standard, just like regex, but with added accuracy.
- Domain and SMTP Verification: Beyond regex, Heybounce goes the extra mile to check if the domain is active and the mailbox is deliverable.
- Reduced Bounce Rates: With verified, clean email lists, you’ll experience lower bounce rates, helping maintain your sender reputation.
- Time Efficiency: Relying solely on regex for email validation requires manual implementation and constant upkeep. Heybounce provides an automated solution, helping B2B teams focus on what matters most—connecting with their audience.
If you’re ready to improve your email verification process, give Heybounce a try today and see the difference comprehensive email verification can make.